Creating a Python Package for UnblockMe Puzzles
Written on
Chapter 1 Introduction to the Package
I developed a Python package specifically for tackling UnblockMe puzzles. You can easily install it via Pip by executing the following command in your terminal:
pip install unblockme
How to Utilize This Package
To start, we must represent the puzzle as a string. Here's an example of how the grid looks:
state = '''
AAAB
CDDBEE
CXXB F
C GHHF
G IF
JJJ I
'''
This setup features a 6x6 grid where different letters signify various blocks. The red block is depicted by "XX," while empty spaces are marked by whitespace characters.
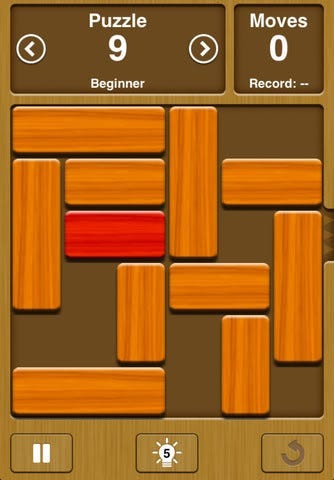
To run the solution, import the package and execute the following code:
from unblockme import unblockme
unblockme(state)
This will animate the solution process in your terminal. Simply hit Enter to progress through each step.
How the Process Works at a Glance
Initially, the state is transformed into a tuple of strings:
state = (
'AAAB ',
'CDDBEE',
'CXXB F',
'C GHHF',
' G IF',
'JJJ I ',
)
Next, we create a function that generates all potential states from the current configuration. For example, the initial state would yield:
# generates
[
(
'AAAB ',
' DDBEE',
'CXXB F',
'C GHHF',
'C G IF',
'JJJ I ',
),
(
'AAAB ',
'CDDBEE',
'CXXB F',
'C GHHF',
' G IF',
' JJJI ',
),
(
'AAAB ',
'CDDBEE',
'CXXB ',
'C GHHF',
' G IF',
'JJJ IF',
)
]
Given that we can derive all possible next states, we can implement Dijkstra’s shortest path algorithm to determine the most efficient route to the goal state.
Example of a Completed State:
GAAA
DDG EE
C XX
CHHB F
C BIF
JJJBIF
Notice that the "XX" (our red block) is positioned on the far right, indicating that it can escape the board.
Conclusion
Enjoy experimenting with the package! Please reach out if you encounter any issues.
I appreciate all your support! Feel free to leave a comment with your thoughts or highlight your favorite parts of this guide.
In this video, "Learn Python by Solving Puzzles," you will find practical examples and insights into enhancing your coding skills through problem-solving.
Watch "Leetcode 3: Longest Substring Without Repeating Characters Part 1" to deepen your understanding of algorithmic challenges and improve your programming expertise.