Implementing Function Overloading in Python: A Comprehensive Guide
Written on
Chapter 1: Understanding Function Overloading
Function overloading is a programming technique often associated with statically-typed languages. However, it is possible to achieve this in Python by utilizing Multiple Dispatch, commonly referred to as multimethods.
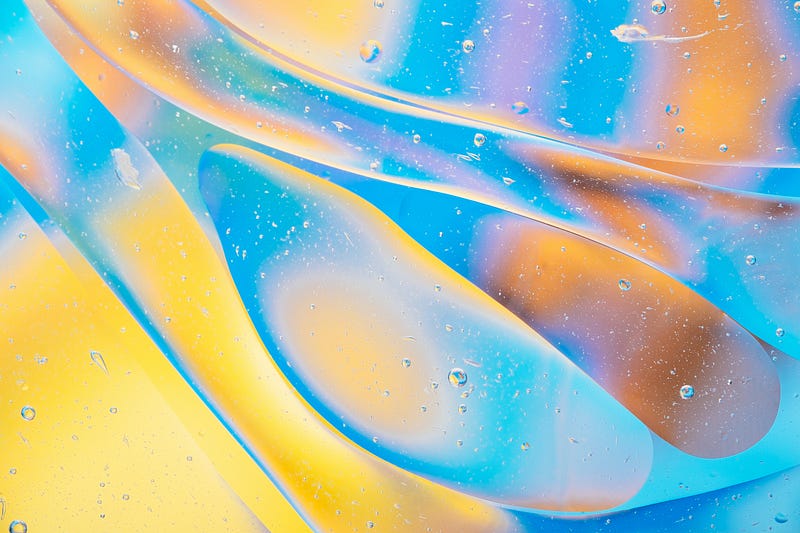
You may have been informed that function overloading cannot be done in Python. The truth is, while Python is dynamically typed and lacks traditional method overloading, we can still implement a similar functionality suited for dynamically-typed languages. This method, known as Multiple Dispatch or multimethods, allows the interpreter to determine which function implementation to use at runtime based on the types of arguments passed.
You might wonder if this approach is necessary. Although traditional overloading isn't feasible, there are compelling reasons to adopt some form of it in Python. Using this technique can enhance code readability, conciseness, and reduce complexity. The alternative, relying on type checks with isinstance(), is often messy and not easily extensible—essentially an anti-pattern.
Moreover, Python already utilizes method overloading for operations such as len() and object instantiation through "dunder" or magic methods, which we frequently use. If we can implement proper overloading for functions, why not embrace that capability?
Chapter 2: Single Dispatch vs. Multiple Dispatch
Now that we understand the concept of overloading in Python, let’s explore how we can implement it effectively.
Single Dispatch
Single Dispatch, as the name suggests, allows overloading based on a single argument type. This feature is available through the singledispatch decorator in the functools module.
To illustrate this, let's look at a practical example of formatting dates and times:
from functools import singledispatch
from datetime import date, datetime, time
@singledispatch
def format(value):
raise NotImplementedError("Unsupported type")
@format.register
def _(d: date):
return d.strftime("%Y-%m-%d")
@format.register
def _(dt: datetime):
return dt.strftime("%Y-%m-%d %H:%M:%S")
@format.register
def _(t: time):
return t.strftime("%H:%M:%S")
In this example, we define a base formatting function decorated with @singledispatch. Then, specific implementations for each type (date, datetime, and time) are registered, enabling the correct function to be called based on the argument's type.
Multiple Dispatch
If your use case requires handling multiple argument types, you’ll need to turn to the multipledispatch library, which can be installed via pip install multipledispatch. This library allows you to define multiple functions sharing the same name but differing in argument types.
from multipledispatch import dispatch
@dispatch(int, int)
def concatenate(a, b):
return str(a) + str(b)
@dispatch(str, str)
def concatenate(a, b):
return a + b
Here, the @dispatch decorator enables us to create different versions of the concatenate function based on the types of its arguments. If you wish to provide a base implementation for unspecified types, you can specify one using @dispatch(object, object).
For more advanced uses, you can incorporate union types or abstract types, such as using Sequence from collections.abc to handle lists, tuples, and ranges seamlessly.
The first video titled "Function Overloading with Python" provides a detailed overview of implementing function overloading techniques effectively in Python.
The second video, "Overloading Functions in Python - @singledispatch," dives deeper into the single dispatch method and its applications.
Closing Thoughts
This discussion has showcased a powerful yet underutilized concept in Python: function overloading through multiple dispatch. Embracing this approach can significantly enhance code clarity and eliminate anti-patterns like type inspection. I encourage you to explore these techniques in your projects to see the benefits firsthand.
For those interested in a more in-depth understanding, consider implementing multimethods yourself, as detailed in Guido van Rossum's article. Additionally, while this article focused on function overloading, many resources cover operator overloading and constructor overloading, which are also valuable topics to explore.