Executing JavaScript Code Stored in a String: A Comprehensive Guide
Written on
Chapter 1: Introduction to Executing JavaScript Code
When working with JavaScript, you might encounter situations where you need to run code stored as a string. This guide explores several methods to execute such code securely and effectively.
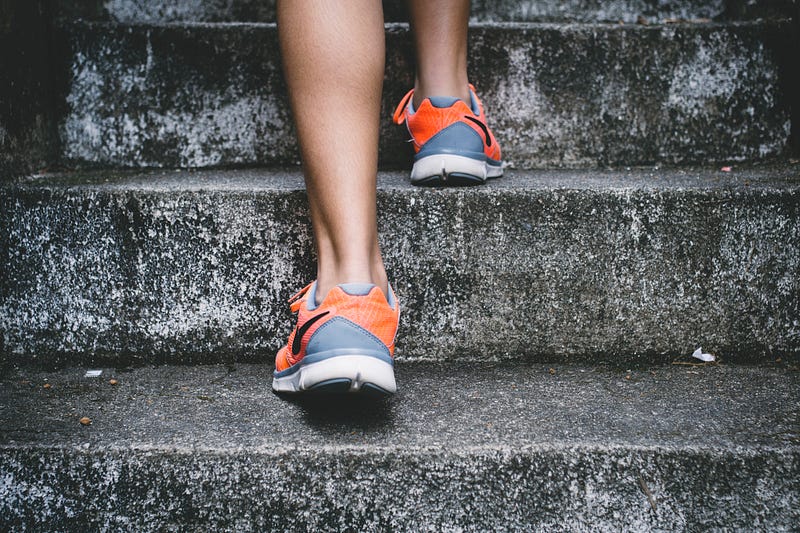
Chapter 2: Creating Functions with the Function Constructor
One effective way to execute JavaScript code stored in a string is by using the Function constructor. This allows you to create a new function dynamically from your code string.
For example, you can define a code string and create a function as follows:
const code = "alert('Hello World'); let x = 100";
const F = new Function(code);
F();
In this snippet, the code string is assigned to the variable code, which is then passed to the Function constructor to generate a function. Calling F() executes the code.
Chapter 3: Utilizing the setTimeout Function
Another method for executing JavaScript code stored in a string is through the setTimeout function. This approach allows you to delay the execution of the code.
Here's an example:
const code = "alert('Hello World'); let x = 100";
setTimeout(code, 1);
In this case, the code string is passed as the first argument to setTimeout, with the second argument defining the delay in milliseconds before execution.
Chapter 4: Avoiding the eval Function
It is crucial to avoid using the eval function when executing code from a string. Using eval poses significant security risks, as it allows for potential code injection.
Additionally, eval creates its own scope, which can lead to unexpected behavior. Therefore, you should refrain from using code like this:
const code = "alert('Hello World'); let x = 100";
eval(code);
Chapter 5: Conclusion
In summary, you can execute JavaScript code stored in a string safely by using the Function constructor or the setTimeout function. Avoid the eval function to protect against security vulnerabilities and unexpected behaviors.
To learn more, visit PlainEnglish.io, and consider subscribing to our free weekly newsletter. Connect with us on Twitter and LinkedIn, and join our Community Discord and Talent Collective.
Chapter 6: Practical Demonstrations
Explore how to execute a JavaScript program directly in your browser with practical examples.
Learn about the best environments and tools for executing JavaScript code in this informative tutorial.