Add Engaging Charts to Your React App with Victory Tooltips
Written on
Chapter 1: Introduction to Victory Charts
Victory provides a robust way to integrate charts and data visualization into your React applications. This guide explores how to incorporate these elements using Victory, focusing on tooltip customization.
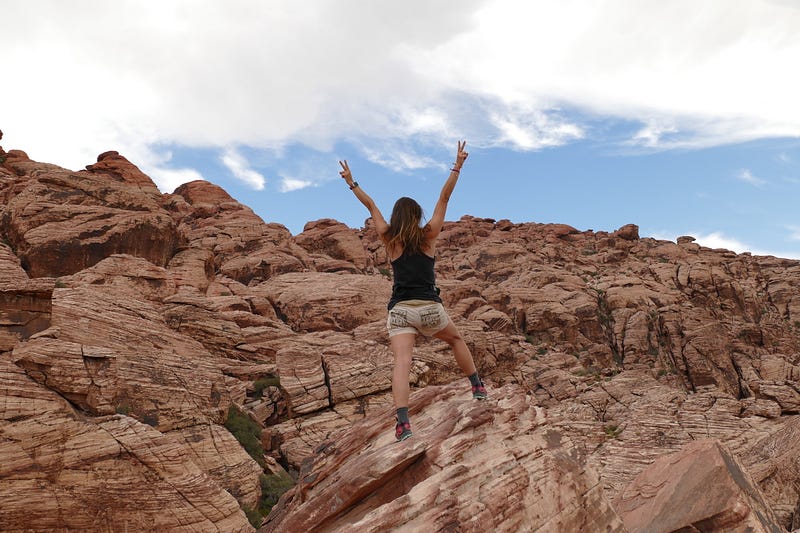
Section 1.1: Adding a Mouse Following Tooltip
One of the standout features of Victory is the ability to implement a tooltip that tracks mouse movements. To achieve this, you can use the following code snippet:
import React from "react";
import {
VictoryAxis,
VictoryChart,
VictoryLine,
VictoryScatter,
VictoryTooltip,
VictoryVoronoiContainer
} from "victory";
export default function App() {
return (
<VictoryChart
domain={{ y: [0, 6] }}
containerComponent={
<VictoryVoronoiContainer
mouseFollowTooltips
voronoiDimension="x"
labels={({ datum }) => y: ${datum.y}}
/>
}
>
<VictoryScatter
style={{ data: { fill: "red" }, labels: { fill: "red" } }}
data={[
{ x: 0, y: 2 },
{ x: 2, y: 3 },
{ x: 4, y: 4 },
{ x: 6, y: 5 }
]}
/>
<VictoryScatter
data={[
{ x: 2, y: 2 },
{ x: 4, y: 3 },
{ x: 6, y: 4 },
{ x: 8, y: 5 }
]}
/>
</VictoryChart>
);
}
By applying the mouseFollowTooltips property to the VictoryVoronoiContainer, we can enable this tracking feature. Moreover, we can handle additional events using the events property.
Section 1.2: Handling Events in Victory Charts
To illustrate how to manage events, consider the following example:
import React from "react";
import { VictoryBar, VictoryChart, VictoryTooltip } from "victory";
export default function App() {
return (
<VictoryChart domain={{ x: [0, 11], y: [-10, 10] }}>
<VictoryBar
labelComponent={<VictoryTooltip />}
data={[
{ x: 2, y: 5, label: "A" },
{ x: 4, y: -6, label: "B" },
{ x: 6, y: 4, label: "C" },
{ x: 8, y: -5, label: "D" },
{ x: 10, y: 7, label: "E" }
]}
style={{
data: { fill: "tomato", width: 20 }}}
events={[
{
target: "data",
eventHandlers: {
onMouseOver: () => {
return [
{
target: "data",
mutation: () => ({ style: { fill: "gold", width: 30 } })
},
{
target: "labels",
mutation: () => ({ active: true })
}
];
},
onMouseOut: () => {
return [
{
target: "data",
mutation: () => {}
},
{
target: "labels",
mutation: () => ({ active: false })
}
];
}
}
}
]}
/>
</VictoryChart>
);
}
Here, we utilize the onMouseOver and onMouseOut properties to manipulate the style of the chart bars when the user hovers over them.
Chapter 2: Creating Custom Tooltips
To implement a unique tooltip, you can create a custom tooltip component like so:
import React from "react";
import { VictoryBar, VictoryChart, VictoryTooltip } from "victory";
const CustomTooltip = (props) => {
const { x, y } = props;
const rotation = rotate(-45 ${x} ${y});
return (
<g transform={rotation}>
<VictoryTooltip {...props} renderInPortal={false} /></g>
);
};
CustomTooltip.defaultEvents = VictoryTooltip.defaultEvents;
export default function App() {
return (
<VictoryChart domain={{ x: [0, 11], y: [-10, 10] }}>
<VictoryBar
labelComponent={<CustomTooltip />}
data={[
{ x: 2, y: 5, label: "A" },
{ x: 4, y: -6, label: "B" },
{ x: 6, y: 4, label: "C" },
{ x: 8, y: -5, label: "D" },
{ x: 10, y: 7, label: "E" }
]}
style={{
data: { fill: "tomato", width: 20 }}}
/>
</VictoryChart>
);
}
For the custom tooltip to function, ensure that you set the defaultEvents property to VictoryTooltip.defaultEvents, which provides the necessary event handlers.
This video illustrates how to create animated bar charts using React Native, Victory Native, and Skia, enhancing the visual appeal of your data representations.
In this video, learn to animate line charts within your React Native app using Victory, providing dynamic and engaging data visualizations.
Conclusion
By utilizing Victory, you can seamlessly integrate mouse-following and custom tooltips into your React charts, significantly enhancing the user experience.